Home
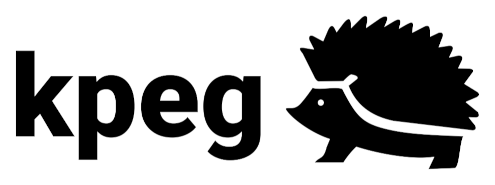

Welcome to the Kotlin PEG parser with Kotlin DSL!
The project is inspired by the pest parser and the kotlin-peg-dsl project.
Simple example
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 | val num = Symbol.rule<Int>(name = "Num", ignoreWS = false) {
seq {
val sign = +char('+', '-').orDefault('+')
val digits = +DIGIT.oneOrMore().joinToString()
value { (sign.get + digits.get).toInt() }
}
}
val sum = Symbol.rule<Int>(name = "Sum") {
num.list(separator = char('+'), min = 1u).mapPe { it.sum() }
}
fun evalExpr(expression: String) =
PegParser.parse(symbol = sum.value(), expression).getOrElse { null }
val results = listOf(
evalExpr("1"), // 1
evalExpr("+1"), // 1
evalExpr("+ 1"), // null
evalExpr("+1 +"), // null
evalExpr("-17"), // -17
evalExpr("-1 7"), // null
evalExpr("1+2+3+4+5"), // 15
evalExpr("1 + +2 + -3 + +4 + 5"), // 9
evalExpr("definitely not expression"), // null
evalExpr(""), // null
)
for (res in results) {
println(res)
}
|
Resources
Things to improve before stable:
TODO
- add more docs
- add more tests, improve the code coverage
- add more built-in characters
- add ability to define
Comment
parsing expression
- better error messages and error handling
- support left recursion
- provide more examples
- multiplatform
Suggestions are welcome!